Movement Acceleration Multiplier
One of the suggestions I got from Guy was to make the player go faster, and so what I did was this, I made the player go progressively faster if they held down the move key, but reset it if they let go.
At the end of the MovePlayer function, I added this AddMovementMultiplier
void AddMovementMultiplier()
{
float newMultiplier = _movementMultiplier += movementMultiplierAmount;
if (newMultiplier > maxMovementMultiplier)
{
return;
}
if (Mathf.Approximately(newMultiplier, maxMovementMultiplier))
{
_movementMultiplier = maxMovementMultiplier;
}
else
{
_movementMultiplier += movementMultiplierAmount;
}
Debug.Log($"movementMultiplier: {_movementMultiplier}");
}
And then when the player stops moving, the _movementMultiplier is set to 0.
I also added a maxMovementMultiplier float to stop the player from going to fast and accelerating to ridiculous, uncontrollable speeds.
Bonus Gravity
Another change I made was an addition of a ForceMultiplier variable. Firstly, I made some changes to the PlayerInput, adding Inputs to accommodate the arrow keys.
Then, I added code in the SelectionArea script. I made a new float called abilityMultiplier and then some functions that interacted with the new Inputs I added.
public void ScrollUp(InputAction.CallbackContext context)
{
if (!context.performed) return;
abilityMultiplier += 1;
Debug.Log($"Scrolling up ability multiplier: {abilityMultiplier}");
}
public void ScrollDown(InputAction.CallbackContext context)
{
if (!context.performed) return;
if (abilityMultiplier > 0)
{
abilityMultiplier -= 1;
}
else
{
abilityMultiplier = 1;
}
Debug.Log($"Scrolling down ability multiplier: {abilityMultiplier}");
}
Then, in PlayerAbility, when the MoveObjectUsingGravity IEnumerator is called, a Vector3 called forceToAdd is made.
objectRb.useGravity = false;
Vector3 forceToAdd = Vector3.up * (radius * _forceAmount * abilityMultiplier);
Debug.Log($"Force to add {forceToAdd}");
objectRb.DOMove(objectRb.transform.position + forceToAdd * Time.fixedDeltaTime, forceMovementTime);
yield return new WaitForSecondsRealtime(forceMovementEnd);
objectRb.useGravity = true;
One of the changes I also made for the gravity ability is that, instead of moving the objects rigidbody, I added a force to the object affected by the ability, and so the DOMove would be replaced with this:
objectRigidBody.AddForce(Vector3.up * radius * _forceAmount, ForceMode.Acceleration);
On Thursday, I provided this summary of what I did to the Discord server:
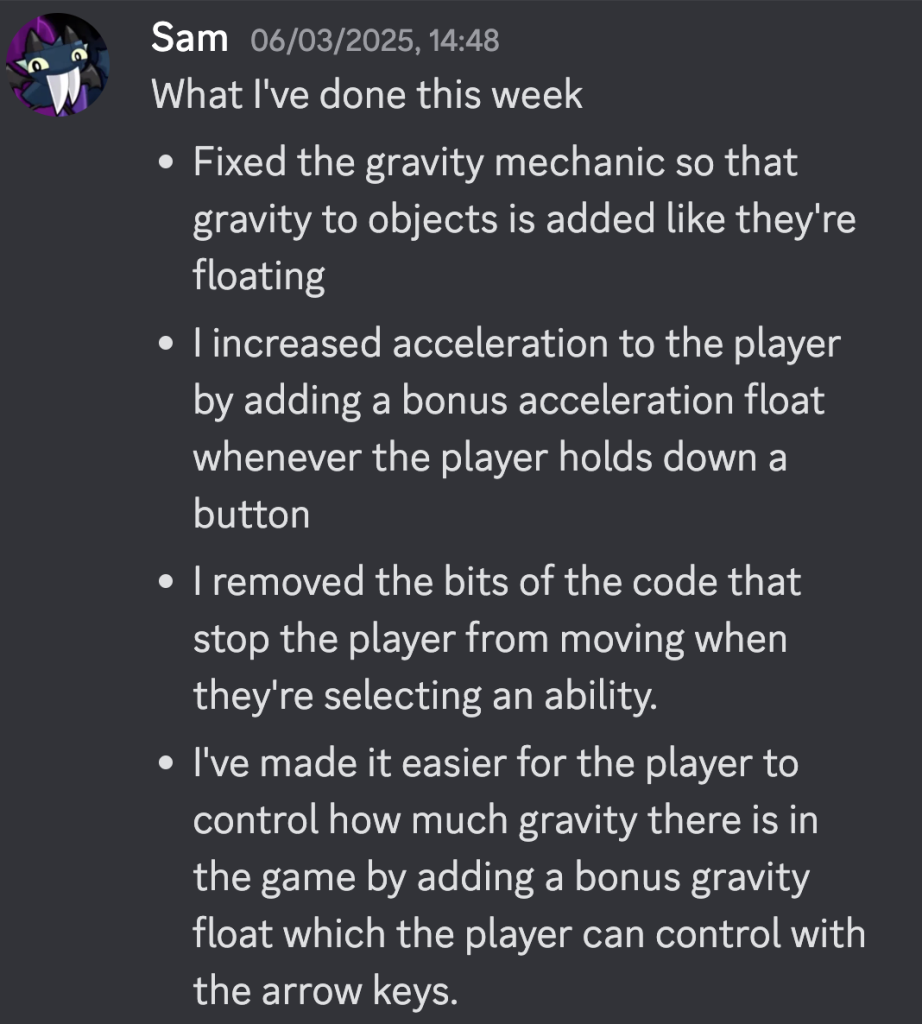