Feedback
Week 7 was reading week, which meant I had no lessons, but I did have a feedback session to attend with Sophie and Vanissa. It was 15 minutes long, and I showed them the work I had done for my blog. They gave me feedback on what to improve with this.
This was the self assessment that Sophie sent to me after the meeting:
Sam Cox
Self assessment:
- Strengths:
- Programming
- To improve:
- Blog
Tutor feedback:
- Move blog to wordpress
- Risk of losing work if you use blogspot
- Some images not appearing
- Good description/ reflection of what happened every week
- Add more categories than just weekly documentation
- What research did you do? Tutorials, programmers in the games industry, etc.
- Talk about how you applied your research to the game – how did it help you improve your ideas?
- Talk about teamwork and communication
- Cross reference your own blog with your team blog
- Add links to show which parts of your work ended up in the final project
- Use this to show how you contributed to the team
- You have done a lot more work than is evident in your blog – it’s important you show us so we can grade you on it
- When possible talk about how your work demonstrates the learning outcomes for the module (you can find the list of them on Blackboard)
We recommend you come to us before Easter and show us your blog after having made changes so we can confirm you’re on track
Assessment Comments
The main thing for me was migrating the content I had created from Blogger to WordPress, although I didn’t get started with this until a few weeks later. Another thing was replacing corrupted images.
Another area for improvement is adding the work I’ve done so far in that week. One way I could do this is by adding references to each of my blog posts where needed in addition to a bibliography page which contains all of the references I’ve used. Finally, I could add sections to the blog posts on how I have improved my communication skills with my teammates.
Game Improvements
I asked Guy what I needed to get done today, he asked for a progress video and I sent it to him:
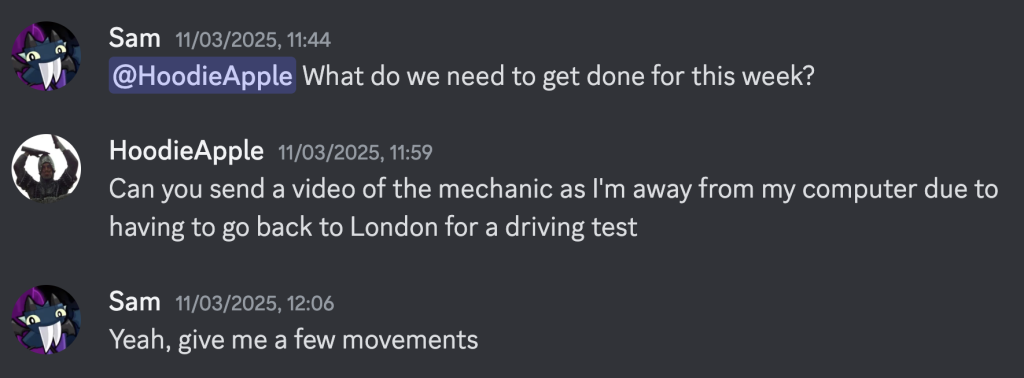
Here’s how he responded:
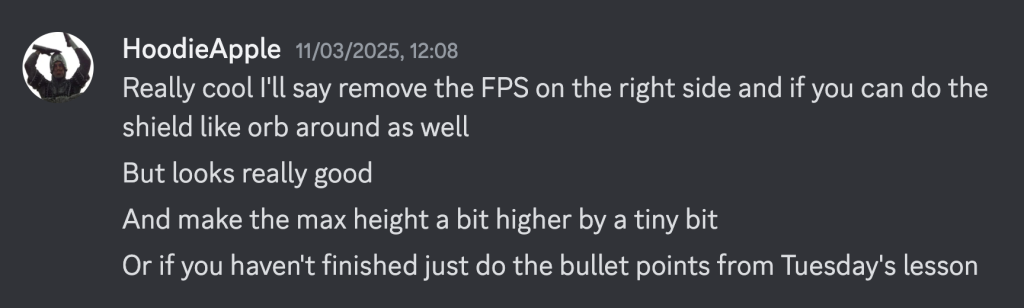
Music
While I had been working on the programming, Esi, while contributing to the art, has also been making some music for the game.
On March 12, she put one of the songs she had been working on in the Discord server.
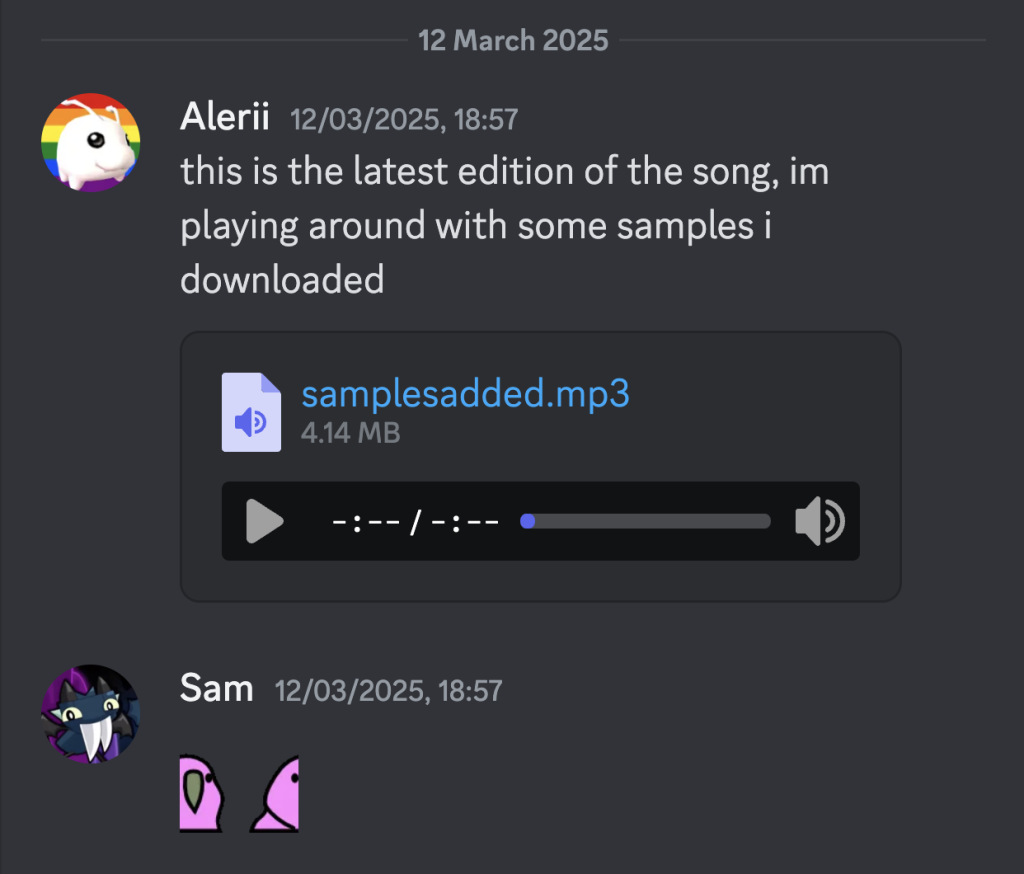
Basic Audio
I then began to integrate this music into the game. One of the first things I did was make a new GameObject (GO) called MusicManager, which, of course, holds the music for the game. Creating a new GO for the music script instead of putting it in the GameManager GO had a benefit of being able to implement Singletons how I wanted, which is what I will go into shortly.
Within the MusicManager GO, I created a new script of the same name where I would store the music file. Esi had exported the music file as a .mp3, which does works in Unity, but from past experience working on a jam game called The Patron, I expect that the file won’t play when using the game in WebGL.
Getting the music to play isn’t a difficult endeavor, all I need to do is add a AudioSource component and then the following code:
[SerializeField] AudioClip[] audioClips;
AudioSource audioSource;
void Start()
{
audioSource = GetComponent<AudioSource>();
audioSource.clip = audioClips[0];
audioSource.Play();
}
The first 2 lines give us references to play with, that being the audio files and the AudioSource component. In the Start function, the AudioSource is cached, its Clip variable is set to the first file in the audioClips array, and then it plays the clip.
One more step to make sure it works, in the AudioSource component, I made sure that the Spatial Blend slider is to the left, to indicate 2D audio, rather than to the right, which would make the audio 3D or based on the environment.
Singletons
If we run this code now, it does work, however one issue is that if the player falls off the map, the game reloads the current scene, resetting the audio.
The way around this is by using a coding technique called a Singleton Pattern. What I am doing is that, when the scene loads, the code checks to see if the same component already exists, if it does, then it destroys itself, if it doesn’t, then it sets itself as the instance.
Implementing this took a bit more research than usual, but here’s the result:
private static MusicManager instance = null;
public static MusicManager Instance
{
get { return instance; }
}
private void Awake()
{
if (instance != null && instance != this) // If another instance exists and is not this one, destroy this.
{
Destroy(gameObject);
return;
}
else // Otherwise, set the instance to this.
{
instance = this;
}
DontDestroyOnLoad(this);
}
I told Esi that I had implemented music into the game and she responded by saying that it was an iterative process.
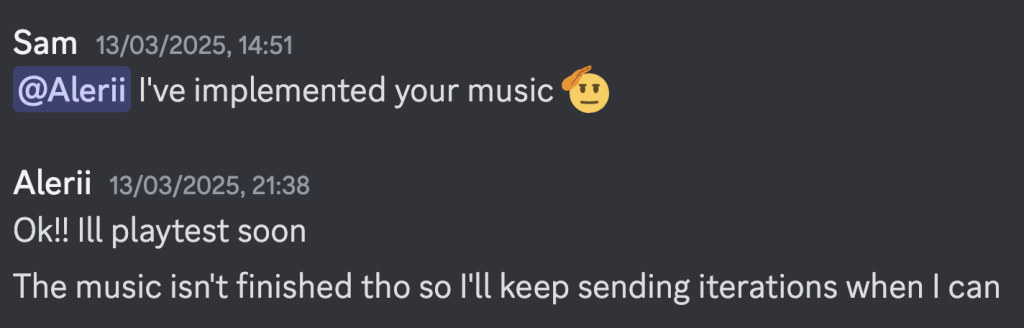
Ability Multiplier
Something else I worked on this week was an ability multiplier, this was intended to be a float that had an effect on the ability that was being casted, for the gravity ability, it could be that the objects get sent higher, and for the possession ability, it could be that the objects move faster.
I started by adding a new Input for the InputAction. It was initially 2 Inputs, ScrollUp and ScrollDown, but with some coding help from Discord for the script, I was later able to merge the 2 into 1.
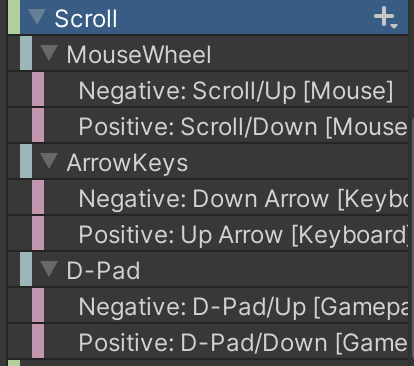
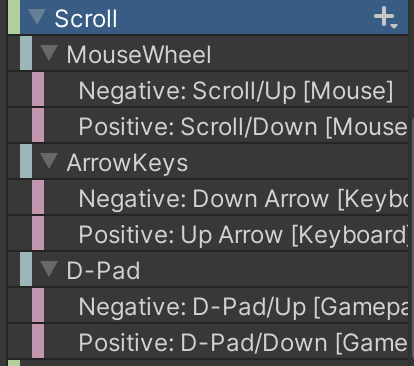
In the PlayerAbility script, I added 2 functions, one that would handle scrolling up, and the other that would handle scrolling down.
void ScrollUp()
{
float newAbility = abilityMultiplier += Time.deltaTime;
if (newAbility > abilityMultiplierMax) return;
abilityMultiplier = newAbility;
Debug.Log($"Scrolling up ability multiplier: {abilityMultiplier}");
}
void ScrollDown()
{
if (abilityMultiplier > 0)
{
abilityMultiplier -= Time.deltaTime;
}
else
{
abilityMultiplier = 1;
}
Debug.Log($"Scrolling down ability multiplier: {abilityMultiplier}");
}
FPS Counter Removal
Finally, following the advice from Guy, I removed the FPS counter from the top left of the screen as it was confusing the other developers and potentially players too.