Movement Improvements
By this point, I had a semi-workable character controller where the player could move the ball around, however, I knew that it was far from where it needed to be in terms of functionality and juice, and so I started to work on improving it.
I started by adding code that would make the ball move in a certain way. At first it didn’t have the intended effect, instead making the player float into the sky, but eventually I was able to get the ball to move while the camera also rotates in a snapping direction. This would be changed later in the process but for now this was what I had.
void MovePlayer()
{
Vector3 movementVelocity = new Vector3(_direction.x, 0, _direction.y);
movementForce = movementVelocity * moveSpeed * Time.fixedDeltaTime;
movementForce.y = 0;
_rb.velocity += movementForce;
RotateCamera(movementVelocity);
}
void RotateCamera(Vector3 rotationVelocity)
{
// Convert rotationVelocity to... something.
float rotationAngle = Mathf.Atan2(rotationVelocity.x, rotationVelocity.y) * Mathf.Rad2Deg;
Debug.Log("Rotation Angle: " + rotationAngle);
// Rotate camera towards rotationVelocity.
CameraFollowObj.DORotate(new Vector3(0, rotationAngle, 0), 0.5f);
}
Camera Fading
One issue that has been present so far is that when the player’s camera goes against a wall, it clips the wall, which makes the camera see the wall and not the player.
To remedy this, I did some research and I found a way to fade out and in of objects, so I created a new script and added this code.
using System;
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using DG.Tweening;
public class CameraObjectAlpha : MonoBehaviour
{
[SerializeField, Range(0.01f, 1f)] float alphaColor = 0.2f;
[SerializeField] LayerMask groundLayer;
[SerializeField] float maxDistance = 10f;
[SerializeField] Transform cameraTransform;
public Color originalAlpha;
[SerializeField] float fadeDuration = 0.2f;
MeshRenderer meshRenderer;
[SerializeField] Color colorCheck;
public List<MeshRenderer> meshRenderers;
void Update()
{
CheckForObjects();
}
void CheckForObjects()
{
Ray objectCheck = new Ray(cameraTransform.position, transform.forward);
RaycastHit hitData;
if (Physics.Raycast(objectCheck, out hitData, maxDistance, groundLayer))
{
Debug.Log("Hit object " + hitData.collider.gameObject);
meshRenderer = hitData.collider.GetComponent<MeshRenderer>();
if (meshRenderer != null)
{
Debug.Log("Mesh Renderer Detected.");
SetObjectAlpha();
}
}
else
{
StartCoroutine(ResetAlpha());
}
}
void OnTriggerEnter(Collider other)
{
if (other.gameObject.CompareTag("World"))
{
meshRenderer = other.gameObject.GetComponent<MeshRenderer>();
}
}
void SetObjectAlpha()
{
meshRenderer.material.DOFade(alphaColor, fadeDuration);
}
IEnumerator ResetAlpha()
{
yield return new WaitForSeconds(fadeDuration);
for (int i = 0; i < meshRenderers.Count; i++)
{
if (meshRenderers[i] != meshRenderer && !Mathf.Approximately(meshRenderers[i].material.color.a, 255))
{
meshRenderers[i].material.DOFade(originalAlpha.a, fadeDuration);
}
}
}
}
It worked relatively well for the time being, however, one issue I had which kept coming up was that, when the objects reset, they would not go back to their original alpha but instead emit a blindingly bright glow, so this script would be removed later on in the process.
Instagram Page
I also decided to create a business Instagram page to advertise the game in the future. I’ve got experience of using Instagram for this, as I made a business Instagram account for my BTEC IT studies. So far it hasn’t been used for promotion of the game but that could change in the future.
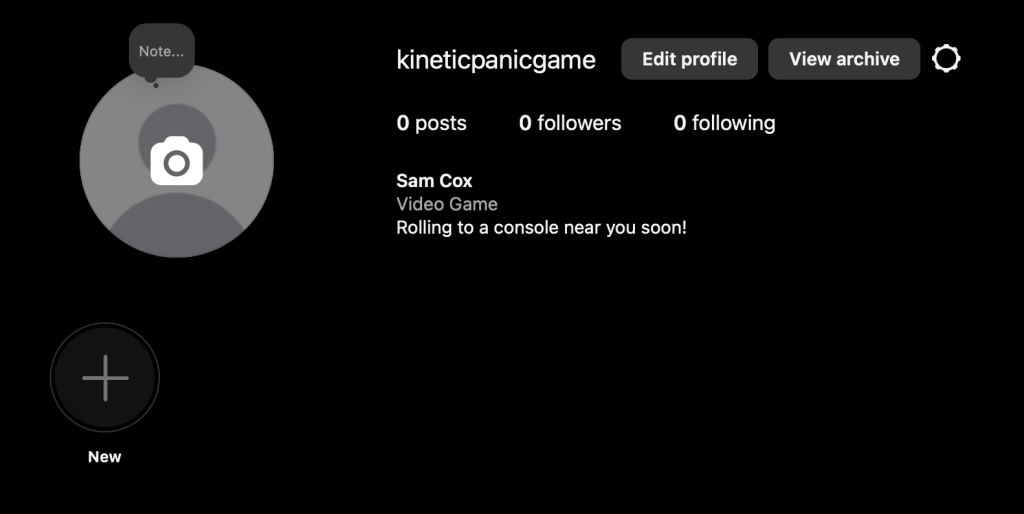
Final Week Camera Changes
At the end of the week, I asked Esi for some feedback on the new version of the prototype.
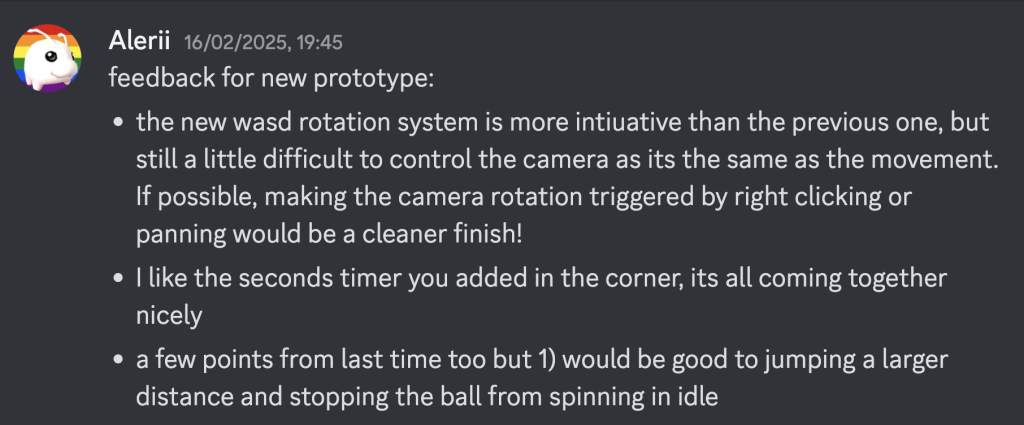
To remedy the first point she made, I added a function in the PlayerMovement script that disabled the camera when the player wasn’t using the input, otherwise it would enable it.
public void OnMoveCamera(InputAction.CallbackContext context)
{
if (context.performed && cinemachineFreeLook.enabled == false)
{
cinemachineFreeLook.enabled = true;
}
else
{
cinemachineFreeLook.enabled = false;
}
}
This would eventually be removed in response to further feedback.