After my research on Anna’s GDD and existing roguelite/ roguelike games and their procedural room generation. I felt I was knowledgeable enough on it to begin its development. To start off, I followed a YouTube tutorial so that I would have a basis that I could expand and build upon once I had finished it.
Prototype 1
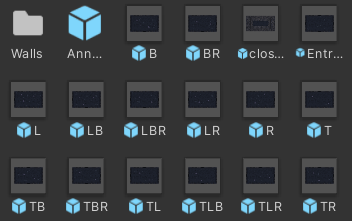
Note: This screenshot was taken later, which is why the rooms already have artwork.
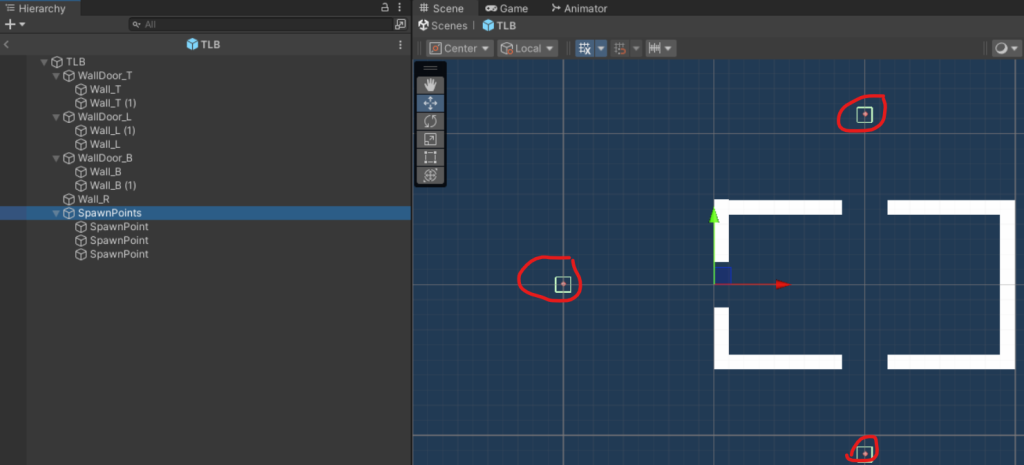
(Has doorways on Top, Left and Bottom)
gameobjects in the red circles being the SpawnPoints, where other room prefabs would be spawned.
How the room generation works is that I created a variety of ‘Room’ prefabs, these room prefabs were all the same heigh and width, with a different amount of doors in different directions to one another. They would then be instantiated next to one another on ‘SpawnPoints’ that were half of a room’s width or height away (Depending on whether the room would spawn beside another or above/below it). This would all start from an ‘EntryRoom’ a room that had four doorways (one in each direction) and therefore four SpawnPoints that would instantiate rooms all around it. This would be the room that the player would find themselves in when they first entered the Depression Realm.
Each of the doorways would require a different type of room, for example, the SpawnPoint above the entry room would require a room that had a BOTTOM doorway, this is to ensure that the two rooms would link to one another. In order to do this, I created a int variable on the SpawnPoints called ‘openingDirection’. This would tell the SpawnPoint which doors they could instantiate depending on their position.
For example, the SpawnPoints that were above the room, would have an ‘openingDirection’ of ‘1’. I could then, depending on this int variable, instantiate rooms from more specific arrays, like an array of all the rooms that have a doorway leading downwards.
The following is the script for the SpawnPoint gameobjects:
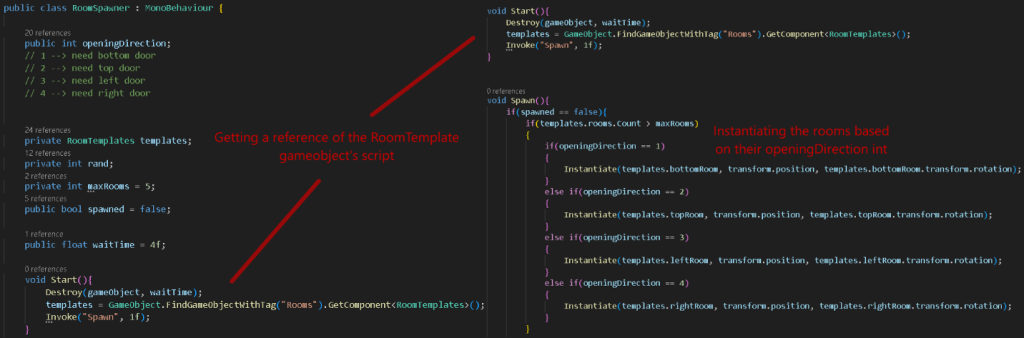
I also created an empty ‘RoomTemplates’ gameobject that would house the arrays that the SpawnPoint gameobjects would choose from to instantiate random rooms.
This is the script for the RoomTemplates gameobject:
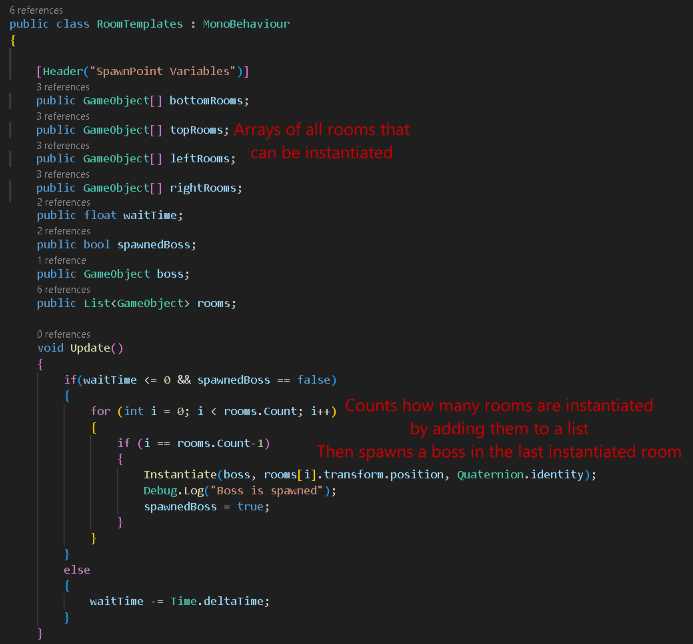
This first prototype of the procedural room generation worked, spawning rooms from an array of prefabs that would link doors from the room that was instantiating the new room, to the new room that was being instantiated. However, there were two main issues that I saw straight away that needed to be fixed (Other than the absurd amount of rooms that were being instantiated).
Issue 1: Rooms were able to instantiate on top of another room that was already there in the first place
Issue 2: Rooms were able to block off other rooms that had doorways leading into them
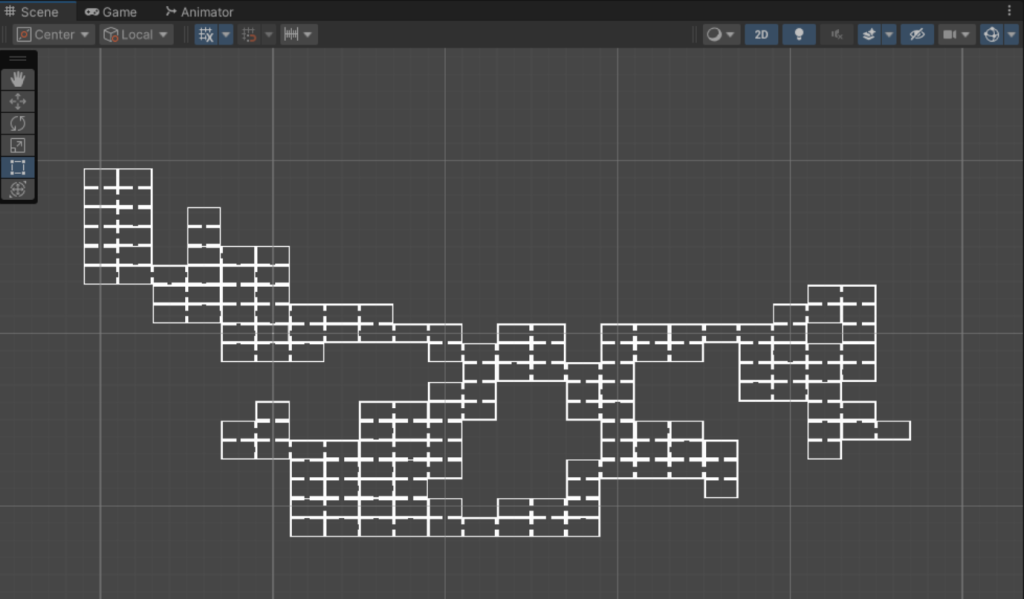
Bug Fixing – 1
In order to fix these bugs, I had to figure out why they were occurring in the first place, for the first issue. I noticed that rooms that were spawned diagonally of one another at the same time (1 & 2), would have SpawnPoints in the same location, causing the to spawn two rooms next to themselves that would collide(3).
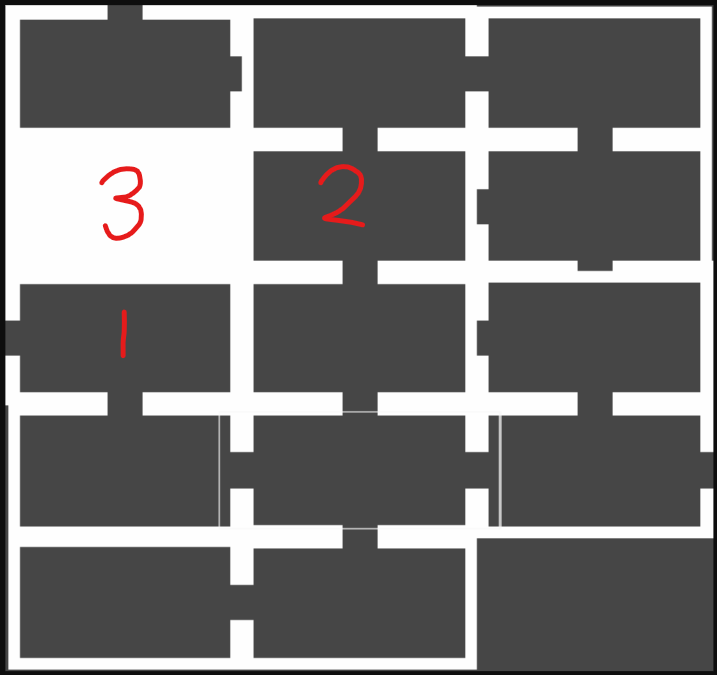
In order to fix this, I created a altered the scripts on the SpawnPoint gameobjects.
I added an OnTriggerEnter2D method to check if the SpawnPoint gameobject collided with another SpawnPoint gameobject. If neither SpawnPoint had instantiated a room, then both SpawnPoints would destroy eachother and place a closedRoom prefab. Effectively closing the room off from all sides.
The following is the addition to the script:
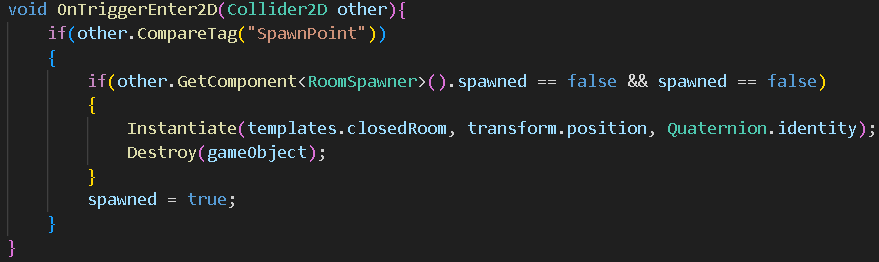
This fixed one instance of the issue of rooms being able to be instantiated on eachother, however, after fixing it. I realised another instance of rooms spawning on eachother as after the fix had been implemented, rooms were still instantiating on top of eachother.
Bug Fixing 2
After finding the bug and I had to pin-point exactly why it was happening. This bug would occur when a SpawnPoint would instantiate inside of a room that already exists there, this SpawnPoint would then spawn a new room, completely unaware of the room that’s already there. I knew this was the case because rooms would spawn in an order, with rooms closer to the entry room being instantiated sooner than rooms further than the entry room.
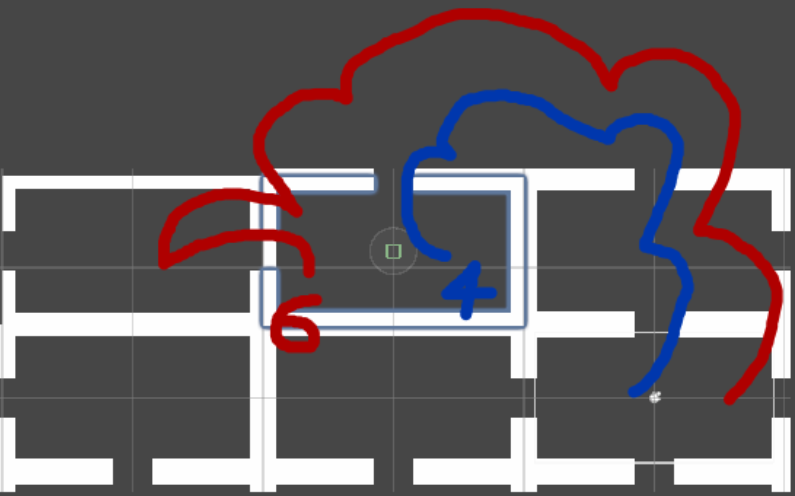
In order to fix this bug, I needed to find a way for the SpawnPoints to check if there was already a room instantiated at the position they were at. In order to do this, I created a gameobject called a ‘Destroyer’ in the middle of every room prefab and gave it a Box Collider 2D (I also gave all of the SpawnPoints a Box Collider 2D). This destroyer would then check everything it collided with, and if it were a SpawnPoint or a closedRoom prefab, it would destroy it:
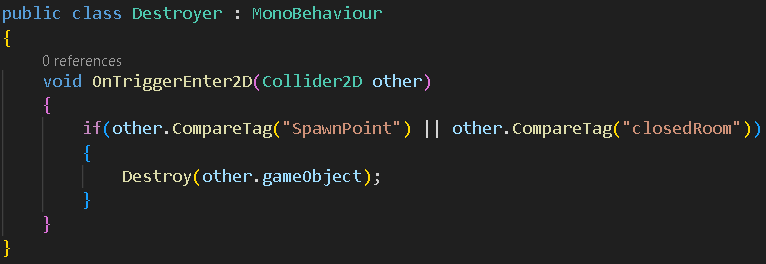
This Destroyer gameobject finally prevented rooms from being able to spawn on top of each other.