Now that the Procedural Room Generation was in a working state, I had to consider how the player would be able to move through and interact with it.
Camera System
When the player moves throughout the dungeons, they need to be able to see the rooms that they are in, in its entirety. Therefore, every time the player enters a room, the camera must lock on to the entire room for two key reasons:
– The player isn’t blind-sided by any attacks that are out of their vision
– Unnecessary space isn’t being taken up in the players’ view
To do this, I needed a way for the game to recognise whenever the player entered a room. So I created an invisible gameobject with a Box Collider 2D; called it a ‘RoomManager’ and added it to every room prefab, to check if the player had entered that room. Then, once the player had entered that room, I needed to find a way for the camera to centre on that room, for whenever the player was colliding with the ‘RoomManager’ game object.
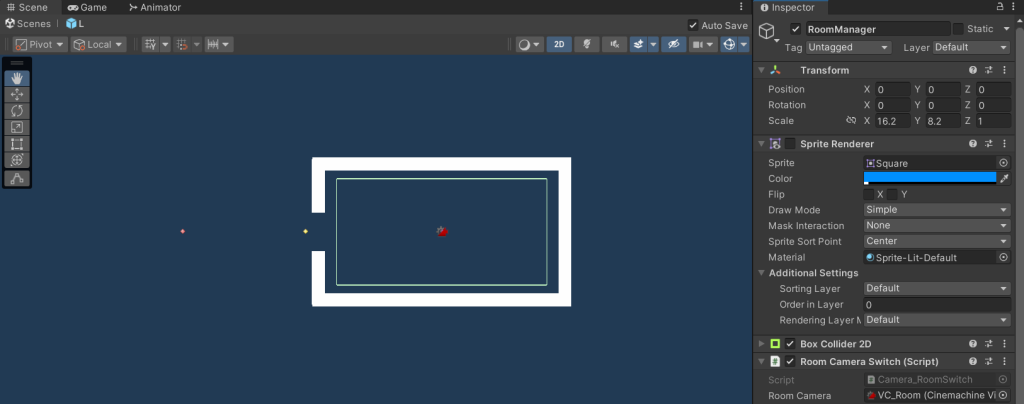
The default camera system in Unity isn’t great, so I downloaded a built-in camera system form the Unity Package Manager called ‘Cinemachine’. Cinemachine allowed me to intuitively create a system for the camera movement between rooms within the dungeon. I then created a Virtual 2D Camera using Cinemachine and implemented one in each room prefab (Ensuring the camera encapsulated the entire room and only the entire room).
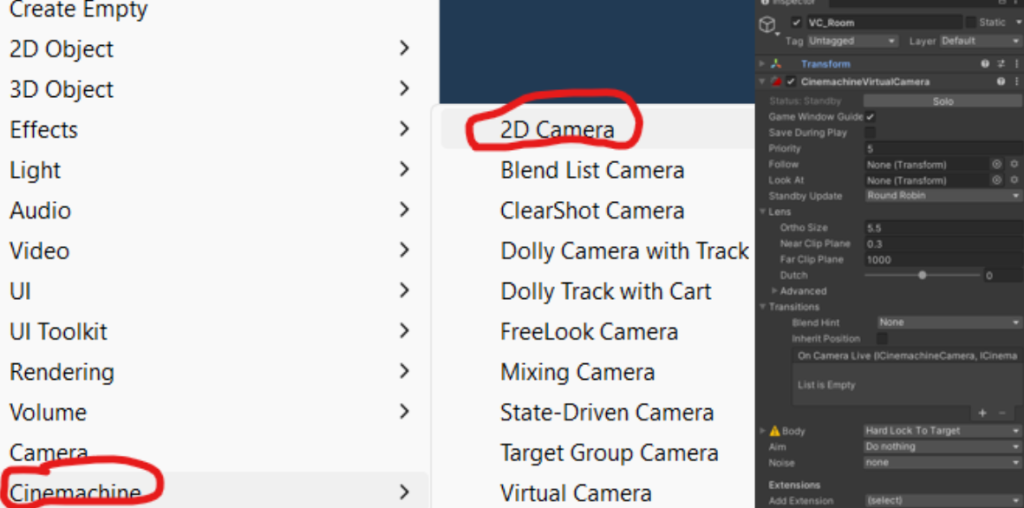
Then, on the ‘RoomManager’ gameobject, I added a ‘RoomCameraSwitch’ script that would essentially swap which VirtualCamera was being used depending on what room the player was in. This was done using the ‘Priority’ integer in the ‘CinemachineVirtualCamera’ component, how the ‘Priority’ int would work is that whatever Virtual Camera had the highest priority int value would be the camera that the game would follow. I had set a Virtual Camera to follow the player and gave it a ‘Priority’ value of 9, and made all of the room prefabs’ Virtual Cameras have a priority of 5. However, whenever the player would enter a room, the ‘Priority’ value of the Virtual Camera of that room would be increased to 10, causing the game to use that room’s camera instead of the player follow camera. Lastly, whenever the player would leave the room (stop colliding with that room’s ‘RoomManager’ gameobject) that room’s Virtual Camera’s Priority value would fall back down to its default value of 5.
The following is the ‘RoomCameraSwitch’ script that is on the ‘RoomManager’ gameobject:
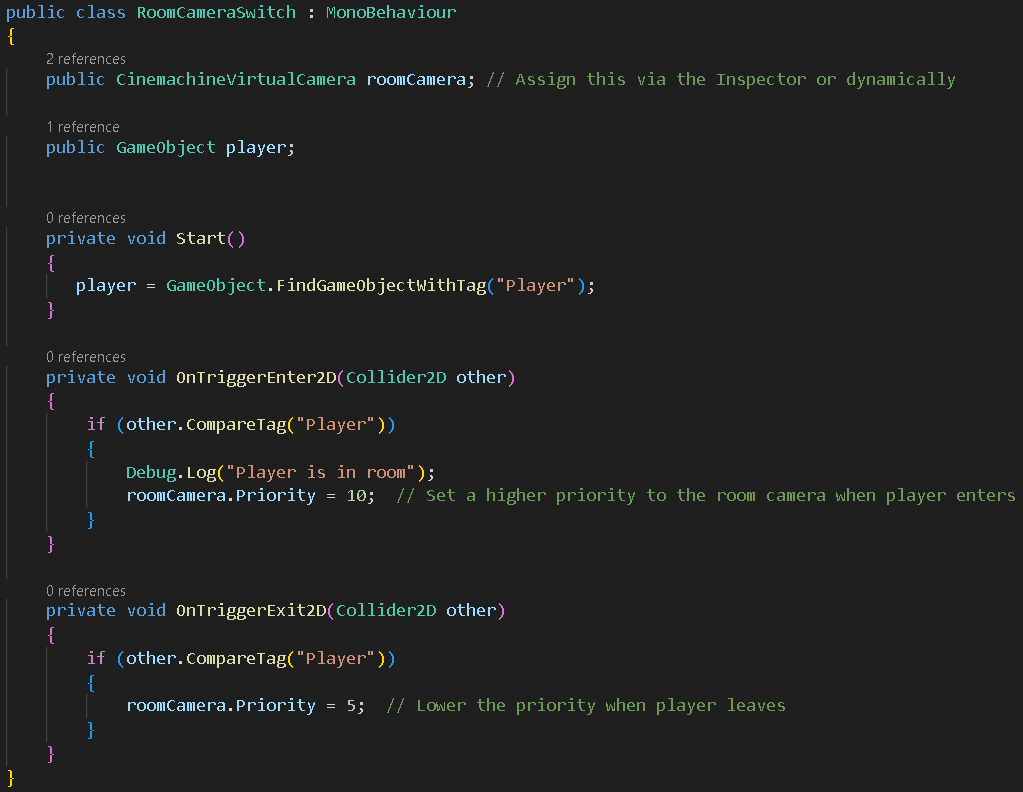
Collisions
In order for the walls to prevent the player from leaving the room before the room was completed, I had to add a Box Collider 2D component to them.
Most importantly, I made sure the ‘Is Trigger’ box was NOT ticked. This would have turned the component into a trigger instead of a collider, allowing the player to completely phase through walls.
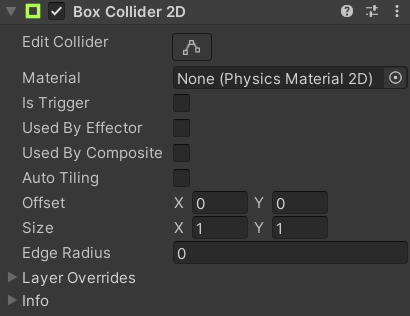