After bug fixing in week 2, the procedural room generation was a lot less buggier. However, although a lot less common, there were still instances of the rooms being able to block one another.
Understanding the Issue
After running the room generation multiple times and observing instances of rooms blocking each other, it became more apparent after comparing these instances to one another.
Usually, RespawnPoints would collide with a SpawnPoint in order to instantiate an appropriate room, however in this instance, the RespawnPoints of rooms 1 & 2 (The yellow numbers) destroyed one another (As the rooms that they came from were instantiated at the same time). Therefore, when room number 3’s SpawnPoint instantiated room number 4, there were no RespawnPoints it could collide with. Resulting in room 3’s SpawnPoint being unable to communicate with room 1 or 2’s RespawnPoints. Which was essentially the same issue that I tried to solve by creating the RespawnPoints in the first place but with an extra step.
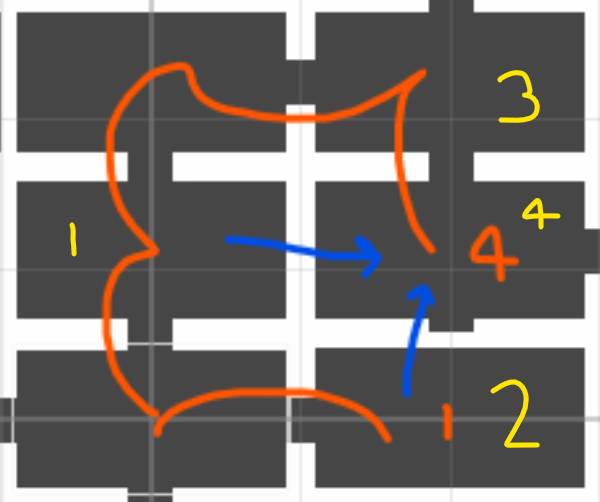
However, I could not fix this issue using the same premise as I did with the RespawnPoints. This was because it would require me to allow RespawnPoints to access other RespawnPoints’ closingDirection ints; which would result in me having to create room arrays for every possible combination of two closingDirections and an openingDirection. Which would result in a total of 64 arrays (4 openingDirections * (4closingDirections)^2). This solution seemed too inefficient and would not only take up a lot of time that wasn’t available (As the playtest was in 2 weeks), but it could also affect the performance of the game, because the number of arrays being accessed by all of these SpawnPoints at the same time was 16 times more than it was during the first prototype of the procedural room generation.
Bug Fixing
However, there was a much simpler and easier way to fix the bug of rooms being able to block each other, this was a solution I had thought of before committing to the previous attempts at fixing the bug, but I had deemed it ‘too easy’ and a ‘cop out’ of sorts. The easy fix was to, instead of being more precise with the rooms that were being instantiated, I could destroy the walls that were blocking other rooms.
Although this way of preventing rooms from blocking one another was avoiding the problem at its source, it was the only way I could think of to create the room generation in time for the playtest (As I had to spend time and resources towards other aspects of the game before the playtest).
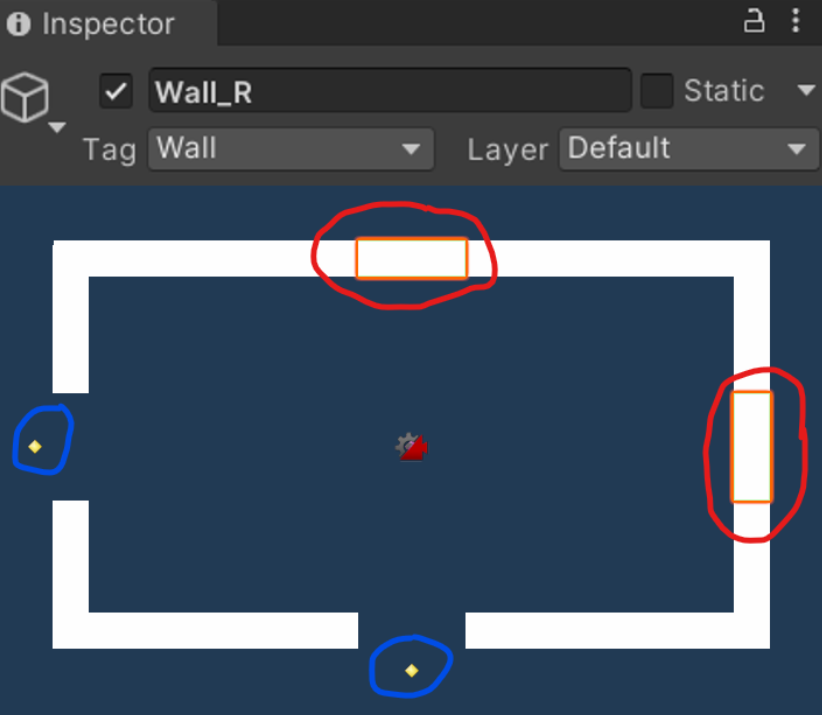
In order to do this, I had to split up the walls without doorways (Circled in red) of each room and assigned them with the tag of ‘Wall’. Then, I created a new gameobject called a ‘DoorDestroyer’ in each of the doorways and placed them where other rooms could block off that rooms doorways. I then gave them scripts that would destroy these walls that blocked them off.
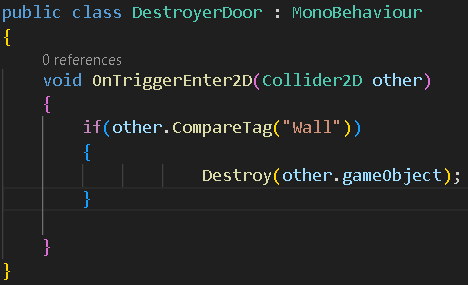
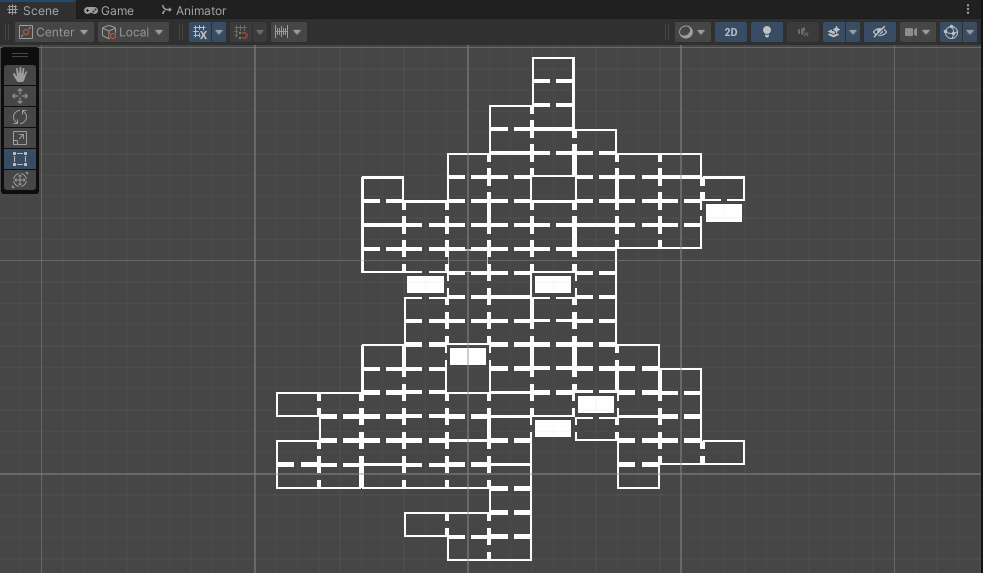
However, there was still the glaring issue of far too many rooms being instantiated inside of the dungeon. According to Anna’s GDD, the first realm the player enters should only have ten rooms total, whereas the current iteration of the procedural room generation would create closer to a hundred.
Decreasing the number of rooms
In order to decrease the number of rooms that get instantiated, I first need to create a way to count how many rooms get instantiated. To do this I created a script called ‘AddRoom’ that adds every room that is instantiated into a list within the ‘RoomTemplate’ script. I then added this script to the EntryRoom as it will always be present in the scene.
The AddRoom script:
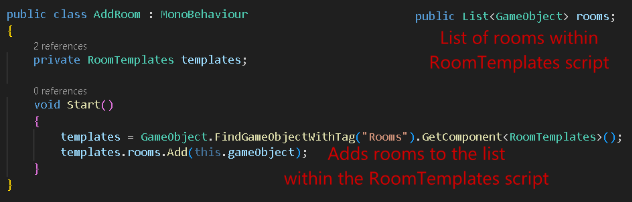
Once I had this list of all instantiated rooms, I needed to access this list from the SpawnPoint scripts, so that those gameobjects knew to change the way they were spawning rooms.
In order to decrease the amount of rooms that were being spawned, I needed the SpawnPoint gameobjects to only spawn rooms with zero exits (Rooms that only had doors that linked to the previous room). To do this, I created public gameobjects in the RoomTemplates scripts that the SpawnPoints would instantiate if the amount of rooms reached a certain number (maxRooms).
The changes to the SpawnPoint script:
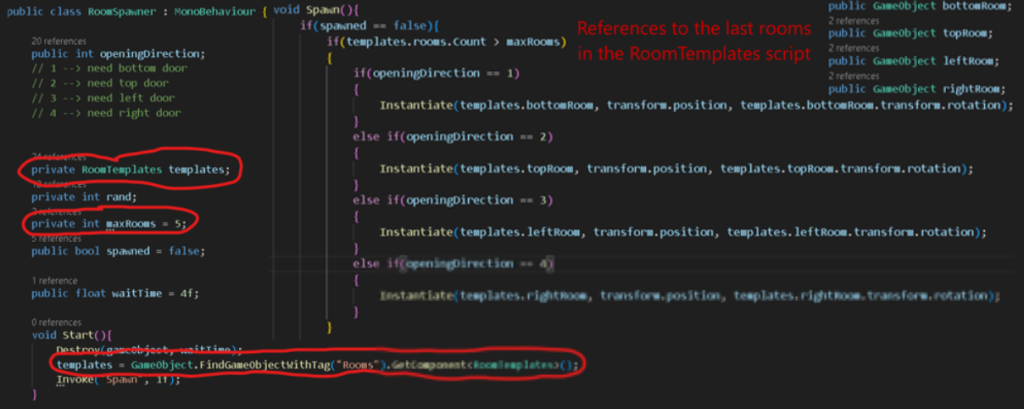
After testing, it became apparent that rooms would never instantiate with exactly 10 rooms everytime. This was because it was still random how many exits needed to be filled when the number of rooms in the dungeon reached or exceeded the maxRooms integer. However, after testing it multiple times, the closest I could get to the dungeon having around 10 rooms was by making the maxRooms int = 5. This would result in the dungeon having 8 – 13 rooms on average, with the average being around 10 rooms.